本章内容将教你如何使用Struts2 应用程序发送电子邮件。学习前,你需要从JavaMail API 1.4.4下载并安装mail.jar,并将mail.jar文件放在WEB-INF\lib文件夹中,然后继续按照以下标准步骤创建action,视图和配置文件。
创建Action
首先是创建一个Action方法来处理电子邮件发送。让我们创建一个包含以下内容的名为Emailer.java的新类:
package cn.w3cschool.struts2; import java.util.Properties; import javax.mail.Message; import javax.mail.PasswordAuthentication; import javax.mail.Session; import javax.mail.Transport; import javax.mail.internet.InternetAddress; import javax.mail.internet.MimeMessage; import com.opensymphony.xwork2.ActionSupport; public class Emailer extends ActionSupport { private String from; private String password; private String to; private String subject; private String body; static Properties properties = new Properties(); static { properties.put("mail.smtp.host", "smtp.gmail.com"); properties.put("mail.smtp.socketFactory.port", "465"); properties.put("mail.smtp.socketFactory.class", "javax.net.ssl.SSLSocketFactory"); properties.put("mail.smtp.auth", "true"); properties.put("mail.smtp.port", "465"); } public String execute() { String ret = SUCCESS; try { Session session = Session.getDefaultInstance(properties, new javax.mail.Authenticator() { protected PasswordAuthentication getPasswordAuthentication() { return new PasswordAuthentication(from, password); }}); Message message = new MimeMessage(session); message.setFrom(new InternetAddress(from)); message.setRecipients(Message.RecipientType.TO, InternetAddress.parse(to)); message.setSubject(subject); message.setText(body); Transport.send(message); } catch(Exception e) { ret = ERROR; e.printStackTrace(); } return ret; } public String getFrom() { return from; } public void setFrom(String from) { this.from = from; } public String getPassword() { return password; } public void setPassword(String password) { this.password = password; } public String getTo() { return to; } public void setTo(String to) { this.to = to; } public String getSubject() { return subject; } public void setSubject(String subject) { this.subject = subject; } public String getBody() { return body; } public void setBody(String body) { this.body = body; } public static Properties getProperties() { return properties; } public static void setProperties(Properties properties) { Emailer.properties = properties; } }
如上面的源代码所示,Emailer.java具有与下面给出的email.jsp页面中的表单属性相对应的属,这些属性分别是:
from - 发件人的电子邮件地址。由于我们使用Google的SMTP,因此我们需要有效的gtalk ID。
password - 上述帐户的密码
to - 发送电子邮件给谁?
Subject - 电子邮件的主题
body - 实际的电子邮件内容
我们没有考虑对上述字段的任何验证,验证将在下一章添加。让我们看看execute()方法, execute()方法使用javax Mail库提供的参数发送电子邮件。如果邮件成功发送,action返回SUCCESS,否则返回ERROR。
创建主页
现行编写主页的JSP文件index.jsp,这将用于收集上面提到的电子邮件相关信息:
<%@ page language="java" contentType="text/html; charset=ISO-8859-1" pageEncoding="ISO-8859-1"%> <%@ taglib prefix="s" uri="/struts-tags"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <title>Email Form</title> </head> <body> <em>The form below uses Google's SMTP server. So you need to enter a gmail username and password </em> <form action="emailer" method="post"> <label for="from">From</label><br/> <input type="text" name="from"/><br/> <label for="password">Password</label><br/> <input type="password" name="password"/><br/> <label for="to">To</label><br/> <input type="text" name="to"/><br/> <label for="subject">Subject</label><br/> <input type="text" name="subject"/><br/> <label for="body">Body</label><br/> <input type="text" name="body"/><br/> <input type="submit" value="Send Email"/> </form> </body> </html>
创建视图
现在创建success.jsp文件,这在action返回SUCCESS结果的情况下会被调用,但如果从action返回ERROR结果,我们将用另一个视图文件。
<%@ page language="java" contentType="text/html; charset=ISO-8859-1" pageEncoding="ISO-8859-1"%> <%@ taglib prefix="s" uri="/struts-tags"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <title>Email Success</title> </head> <body> Your email to <s:property value="to"/> was sent successfully. </body> </html>
以下将是在action返回ERROR的情况下调用的视图文件error.jsp。
<%@ page language="java" contentType="text/html; charset=ISO-8859-1" pageEncoding="ISO-8859-1"%> <%@ taglib prefix="s" uri="/struts-tags"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <title>Email Error</title> </head> <body> There is a problem sending your email to <s:property value="to"/>. </body> </html>
配置文件
最后,让我们使用struts.xml配置文件将所有内容放在一起,如下所示:
<?xml version="1.0" encoding="UTF-8"?> <!DOCTYPE struts PUBLIC "-//Apache Software Foundation//DTD Struts Configuration 2.0//EN" "http://struts.apache.org/dtds/struts-2.0.dtd"> <struts> <constant name="struts.devMode" value="true" /> <package name="helloworld" extends="struts-default"> <action name="emailer" class="cn.w3cschool.struts2.Emailer" method="execute"> <result name="success">/success.jsp</result> <result name="error">/error.jsp</result> </action> </package> </struts>
以下是web.xml文件的内容:
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xmlns:web="http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" id="WebApp_ID" version="3.0"> <display-name>Struts 2</display-name> <welcome-file-list> <welcome-file>index.jsp</welcome-file> </welcome-file-list> <filter> <filter-name>struts2</filter-name> <filter-class> org.apache.struts2.dispatcher.FilterDispatcher </filter-class> </filter> <filter-mapping> <filter-name>struts2</filter-name> <url-pattern>/*</url-pattern> </filter-mapping> </web-app>
现在,右键单击项目名称,然后单击“Export”> “WAR File”以创建WAR文件。然后在Tomcat的webapps目录中部署WAR文件。最后,启动Tomcat服务器并尝试访问URL http://localhost:8080/HelloWorldStruts2/index.jsp,将显示以下界面:
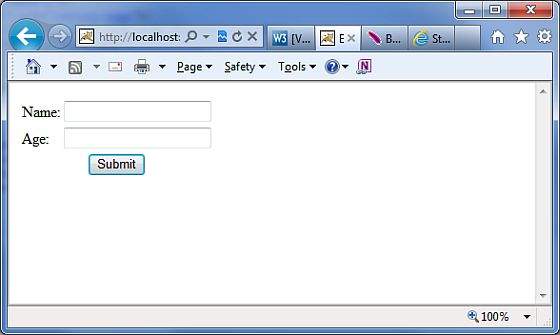
输入所需的信息,然后单击Send Email按钮。如果一切正常,那么你可以看到以下页面:
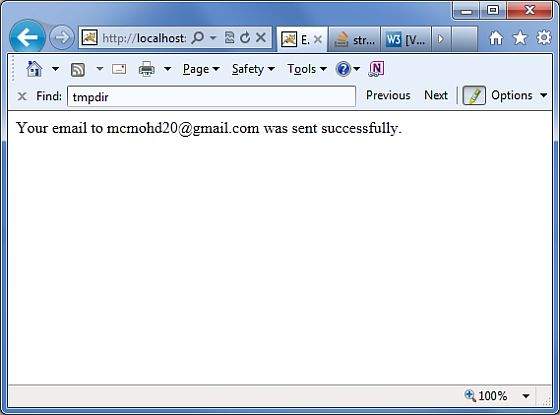
更多建议: