C 结构体
学习C - C结构体
我们可以定义一个struct来声明一个新的数据类型。
我们使用 struct
关键字。
使用struct关键字可以定义一个称为单个单元的结构的各种类型的变量集合。
以下代码是结构声明的简单示例:
struct Dog { int age; int height; } aDog;
此示例声明一个名为Dog的结构类型。
这不是一个变量名;这是一种新的类型。
此类型名称称为结构标签或标签名称。
Dog结构,年龄和高度中的变量名称称为成员或字段。
结构的成员出现在跟随struct标签名称Dog的大括号之间。
在该示例中,结构的实例(称为aDog)被声明。
aDog是Dog类型的变量。
aDog包括结构的两个成员:成员年龄和成员身高。
以下代码为结构类型Dog添加更多成员。
struct Dog { int age; int height; char name[20]; char father[20]; char mother[20]; } aDog = { 4, 1, "name", "C", "C++" };
这个版本有五个成员的狗结构类型。
在变量aDog的声明中,出现在最后一对大括号之间的值按顺序应用,到成员变量age(4),height(1),name(“name"),father(“C")和mother(“C ++")。
定义结构类型和结构变量
您可以在单独的语句中定义结构类型的结构类型和变量。
struct Dog { int age; int height; char name[20]; char father[20]; char mother[20]; }; struct Dog aDog = { 4, 1,"name", "C", "C++" };
第一个语句定义结构标签Dog,第二个是该类型的一个变量的声明,aDog。
以下代码定义了Dog类型的另一个变量:
struct Dog brother = { 3, 5, "new name", "C", "C++" };
您可以在单个语句中声明多个结构变量。
struct Dog aDog, brother;
您可以在单个语句中声明多个结构变量。...
您可以使用typedef定义删除struct。
例如:
typedef struct Dog Dog;
这定义了Dog等同于struct Dog。
如果将此定义放在源文件的开头,你可以定义一个Dog类型的变量,像这样:
Dog t = { 3, 5, "new name", "C", "C++" };
struct关键字不再需要。
访问结构成员
通过写变量名称后跟一个句点来引用结构的成员,其次是会员变量名。
aDog.age = 12;
该期间称为成员选择运算符。
此语句将aDog引用的结构的age成员设置为12。
您可以选择在初始化列表中指定成员名称,如下所示:
Dog t = { .height = 5, .age = 3, .name = "name", .mother = "C", .father = "C++" };
以下代码显示如何定义结构类型,并分配从键盘读取的值以存储在其中。
#include <stdio.h>
typedef struct Dog Dog; // Define Dog as a type name
struct Dog // Structure type definition
{
int age;
int height;
char name[20];
char father[20];
char mother[20];
};
int main(void) {
Dog my_Dog; // Structure variable declaration
// Initialize the structure variable from input data
printf("Enter the name of the Dog: " );
scanf("%s", my_Dog.name, sizeof(my_Dog.name)); // Read the name
printf("How old is %s? ", my_Dog.name );
scanf("%d", &my_Dog.age ); // Read the age
printf("How high is %s ( in hands )? ", my_Dog.name );
scanf("%d", &my_Dog.height ); // Read the height
printf("Who is %s"s father? ", my_Dog.name );
scanf("%s", my_Dog.father, sizeof(my_Dog.father));
printf("Who is %s"s mother? ", my_Dog.name );
scanf("%s", my_Dog.mother, sizeof(my_Dog.mother));
// Now tell them what we know
printf("%s is %d years old, %d hands high,", my_Dog.name, my_Dog.age, my_Dog.height);
printf(" and has %s and %s as parents.\n", my_Dog.father, my_Dog.mother);
return 0;
}
上面的代码生成以下结果。
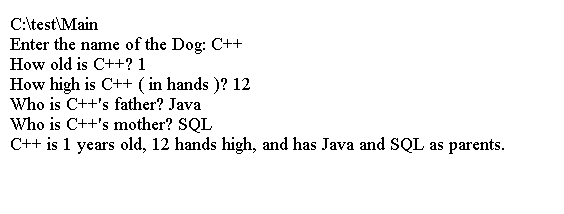
例子
为了说明,我们定义新的数据类型,称为employee。
#include <stdio.h>
// define a struct
struct employee{
int id;
char name[10];
char country[5];
};
int main() {
// declare struct variable
struct employee emp;
// set values
emp.id = 10;
sprintf(emp.name,"jane");
sprintf(emp.country,"DE");
// display
printf("id: %d, name: %s, country : %s\n",emp.id,emp.name,emp.country);
return 0;
}
上面的代码生成以下结果。

结构数组
以下代码显示如何创建结构数组。
#include <stdio.h>
#include <ctype.h>
typedef struct Dog Dog; // Define Dog as a type name
struct Dog // Structure type definition
{
int age;
int height;
char name[20];
char father[20];
char mother[20];
};
int main(void)
{
Dog my_Dogs[3]; // Array of Dog elements
int hcount = 0; // Count of the number of Dogs
char test = "\0"; // Test value for ending
for(hcount = 0 ; hcount < sizeof(my_Dogs)/ sizeof(Dog) ; ++hcount)
{
printf("Do you want to enter details of a%s Dog (Y or N)? ", hcount ? "nother" : "" );
scanf(" %c", &test, sizeof(test));
if(tolower(test) == "n")
break;
printf("Enter the name of the Dog: " );
scanf("%s", my_Dogs[hcount].name, sizeof(my_Dogs[hcount].name));
printf("How old is %s? ", my_Dogs[hcount].name );
scanf("%d", &my_Dogs[hcount].age);
printf("How high is %s ( in hands )? ", my_Dogs[hcount].name);
scanf("%d", &my_Dogs[hcount].height);
printf("Who is %s"s father? ", my_Dogs[hcount].name);
scanf("%s", my_Dogs[hcount].father, sizeof(my_Dogs[hcount].father));
printf("Who is %s"s mother? ", my_Dogs[hcount].name);
scanf("%s", my_Dogs[hcount].mother, sizeof(my_Dogs[hcount].mother));
}
// Now tell them what we know.
printf("\n");
for (int i = 0 ; i < hcount ; ++i) {
printf("%s is %d years old, %d hands high,", my_Dogs[i].name, my_Dogs[i].age, my_Dogs[i].height);
printf(" and has %s and %s as parents.\n", my_Dogs[i].father, my_Dogs[i].mother);
}
return 0;
}
上面的代码生成以下结果。
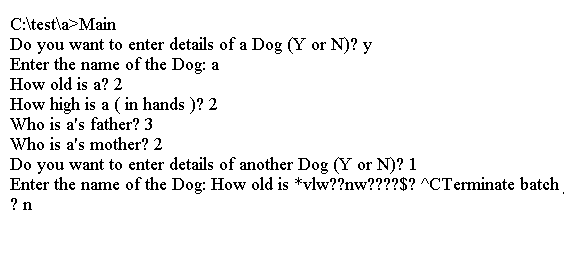
更多建议: